CSS Transition Position Fixed Animation Effect
In this tutorial, we will learn how to apply a CSS transition effect to an element when its fixed position is moved on the page.
Just for reference the end result will work something along these lines:
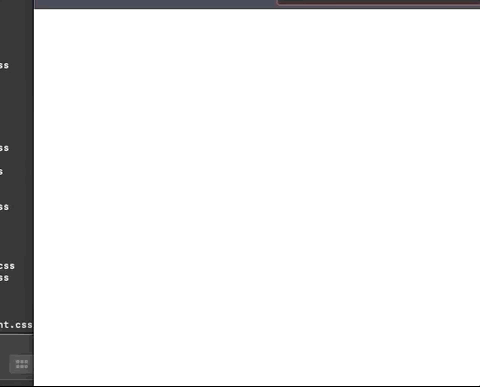
The HTML
The HTML for this simple example will be a box with a nested element. When the outer element is clicked the position of the inner item will change using a transition effect.
<div id="box">
<div class="inner-box"></div>
</div>
Toggle an Active Class with JavaScript
To move the element we will look for a click event on it, then toggle an active class with different positioning rules.
var box = document.getElementById('box');
box.addEventListener('click', function() {
if (box.classList.contains('box-active')) {
box.classList.remove('box-active');
} else {
box.classList.add('box-active');
}
});
Here is a jQuery variant of the same function:
$('#box').click(function() {
$('#box').toggleClass('box-active');
});
The CSS
The CSS will position the inner-box element absolutely outside of the parent element to the left. When .box-active
is added a new position: left
rule is applied with a transition linear effect lasting 2 seconds.
#box {
position: relative;
overflow: hidden;
width: 600px;
height: 400px;
}
.inner-box {
position: absolute;
left: -100px;
top: 100px;
width: 100px;
height: 100px;
background: red;
}
.box-active .inner-box {
left: 100px;
transition: left 0.2s linear;
}