How to Print Coloured Text in Python
When printing the output of a Python program to the terminal it may make it easier to read if we change the color of certain parts of the string. We can approach this in a number of different ways and use packages to make the process easier, which is what we will be learning in this tutorial.
Using ANSI Escape Sequences
The easiest way to print colored text from a Python program is to use ANSI escape sequences. To do this we will create a class containing properties for applying different colors to text. Let's create a file called colors.py
containing a colors class, import that into the program then use it on an f string.
class bcolors:
HEADER = '\033[95m'
OKBLUE = '\033[94m'
OKGREEN = '\033[90m'
WARNING = '\033[93m'
FAIL = '\033[91m'
ENDC = '\033[0m'
BOLD = '\033[1m'
UNDERLINE = '\033[4m'
from colors import bcolors
return f'Successfully downloaded {bcolors.OKGREEN}{file.title}{bcolors.ENDC} to {formt}'
The color of the text is changed between bcolors.OKGREEN
and bcolors.ENDC
:
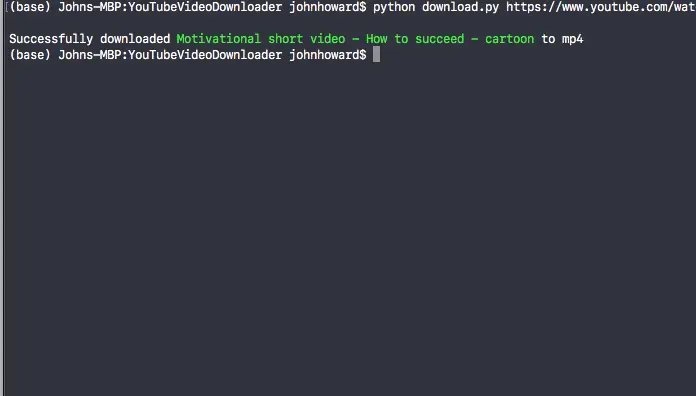
As we can see each ANSI character escape sequence takes the form \033[XXXm
. The part after the [
(square bracket) is the style of the text. Numbers 90-96
provide a range of different text colors. Here are what they are:
Number | Color |
---|---|
90 | grey |
91 | red |
92 | green |
93 | yellow |
94 | blue |
95 | pink |
96 | turquoise |
Personally, I like this approach because you can define any font effect in the class then use it in your program.
Changing the Background Color of Text
To change the background color of text we can use the ANSI escape sequence character numbers 100-107
. Let's expand the bcolors
class we just created to include background colors.
class bcolors:
HEADER = '\033[95m'
OKBLUE = '\033[94m'
OKGREEN = '\033[92m'
WARNING = '\033[93m'
FAIL = '\033[91m'
ENDC = '\033[0m'
BOLD = '\033[1m'
UNDERLINE = '\033[4m'
# Background colors:
GREYBG = '\033[100m'
REDBG = '\033[101m'
GREENBG = '\033[102m'
YELLOWBG = '\033[103m'
BLUEBG = '\033[104m'
PINKBG = '\033[105m'
CYANBG = '\033[106m'
from colors import bcolors
return f'Successfully downloaded {bcolors.GREENBG}{file.title}{bcolors.ENDC} to {formt}'
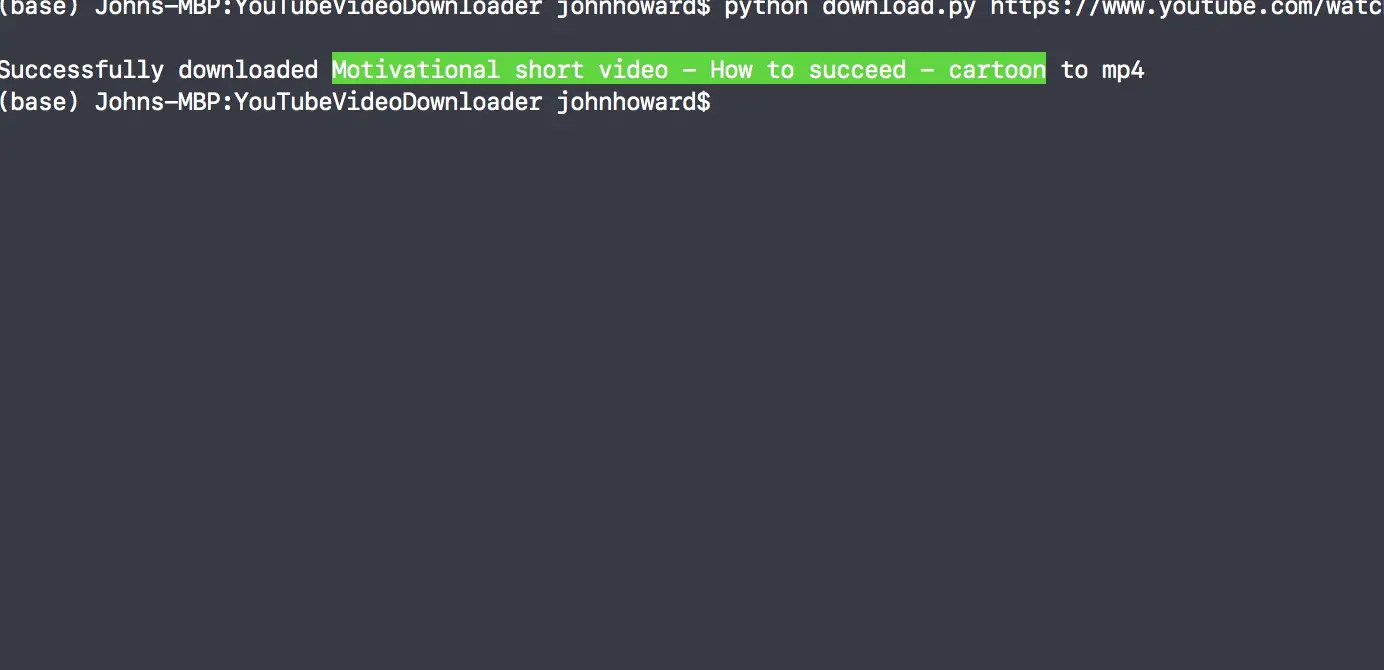
Here are all the available background colors and their corresponding ANSI number:
Number | Color |
---|---|
100 | grey |
101 | red |
102 | green |
103 | yellow |
104 | blue |
105 | pink |
106 | turquoise |
All the Available Font Effects
For reference here are all the available font effect codes to change the style of text in the terminal:
Number(s) | Effect |
---|---|
0 | Reset / Normal |
1 | Bold or increased intensity |
2 | Faint (decreased intensity) |
3 | Italic |
4 | Underline |
5 | Slow Blink |
6 | Rapid Blink |
7 | [[reverse video]] |
8 | Conceal |
9 | Crossed-out |
10 | Primary(default) font |
11–19 | Alternate font |
20 | Fraktur |
21 | Bold off or Double Underline |
22 | Normal color or intensity |
23 | Not italic, not Fraktur |
24 | Underline off |
25 | Blink off |
27 | Inverse off |
28 | Reveal |
29 | Not crossed out |
30–37 | Set foreground color |
38 | Set foreground color |
39 | Default foreground color |
40–47 | Set background color |
48 | Set background color |
49 | Default background color |
51 | Framed |
52 | Encircled |
53 | Overlined |
54 | Not framed or encircled |
55 | Not overlined |
60 | ideogram underline |
61 | ideogram double underline |
62 | ideogram overline |
63 | ideogram double overline |
64 | ideogram stress marking |
65 | ideogram attributes off |
90–97 | Set bright foreground color |
100–107 | Set bright background color |
The colorama Package
If you would rather use a package to change the print colors in Python, install the colorama
package. To install it type the following into your terminal:
pip install colorama
Then import the foreground, background and style classes into your program:
from colorama import Fore, Back, Style
To change some text to red use the Fore.RED
and to set the rest of the text back to normal use Style.RESET_ALL
return f'Successfully downloaded {Fore.RED}{file.title}{Style.RESET_ALL} to {formt}'
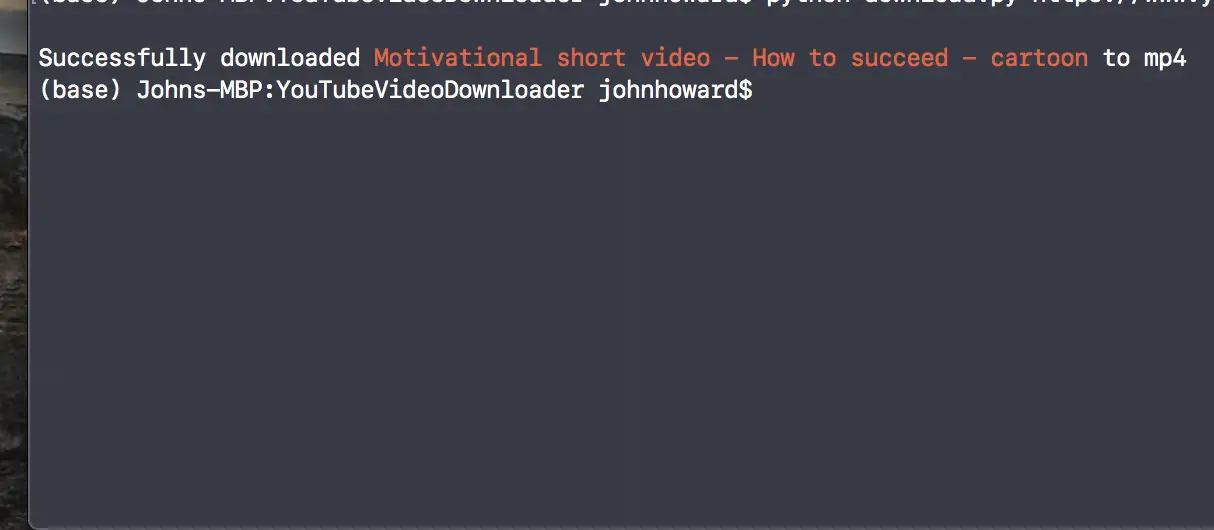
Here are all the formatters available in colorama
:
Fore
- BLACK, RED, GREEN, YELLOW, BLUE, MAGENTA, CYAN, WHITE, RESET.Back
- BLACK, RED, GREEN, YELLOW, BLUE, MAGENTA, CYAN, WHITE, RESET.Style
- DIM, NORMAL, BRIGHT, RESET_ALL
Conclusion
You now know how to style the color text for printing in Python.