Convert Image to Grayscale in Python
Grayscaling is the process of converting an image with a color space such as RGB to just having different shades of grey for each pixel. In this tutorial, we will learn how to grayscale an image in Python.
Convert an Image to Grayscale Using image.convert() From the Pillow Package
The image.convert()
function from the Python Pillow package converts images into different color modes. The mode for 8-bit grayscale is L
and 1
is 1-bit. Here is a full example of converting an image to black and white using Pillow in Python:
from PIL import Image
img = Image.open('/path/to/image.jpg')
img_gray = img.convert('L')
img_gray.save('path/to/image_gray.jpg')
Original image:
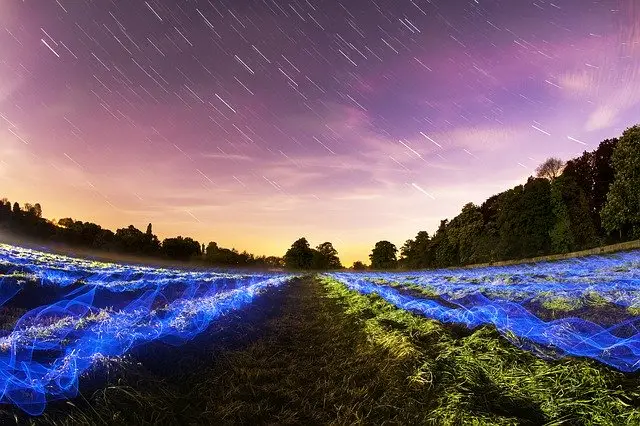
Grayscale image output:
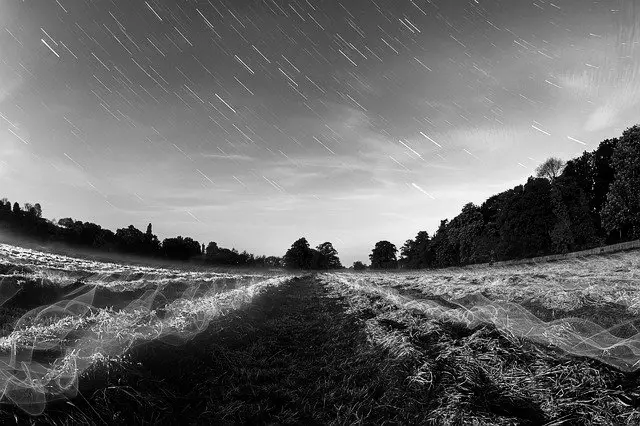
To retain the alpha channel set the mode to LA
:
img_gray = img.convert('LA')
Convert an Image to Grayscale Using color.rgb2gray() From the scikit-image Package
The Python scikit-image package has the built-in color.rgb2gray()
function for converting images to grayscale. To use it import the skimage io
and color
classes, read the image then convert it like this:
from skimage import color
from skimage import io
img = io.imread('image.jpg')
img_gray = color.rgb2gray(img)
Convert an Image to Grayscale Using cv2.imread() From the OpenCV Package
We can read only the grayscale data directly from the original image using the imread()
and setting the second argument (mode) to 0
:
import cv2
img_gray = cv2.imread('image.jpg',0)