How to Generate an Image with Text Programmatically
In this tutorial, we will learn how to generate images with text on them from the command line and from within a PHP program. For this, we will be using a tool from the ImageMagick image processing package rather than FFmpeg. Please make sure you have ImageMagick installed on your system before proceeding.
Generate an Image From the Command Line
To generate an image from the command line line, use the covert
command with the following options:
convert -background "#000000" -size 800x480 -fill "#ffffff" -pointsize 72 -gravity center label:'Some Text' output.png
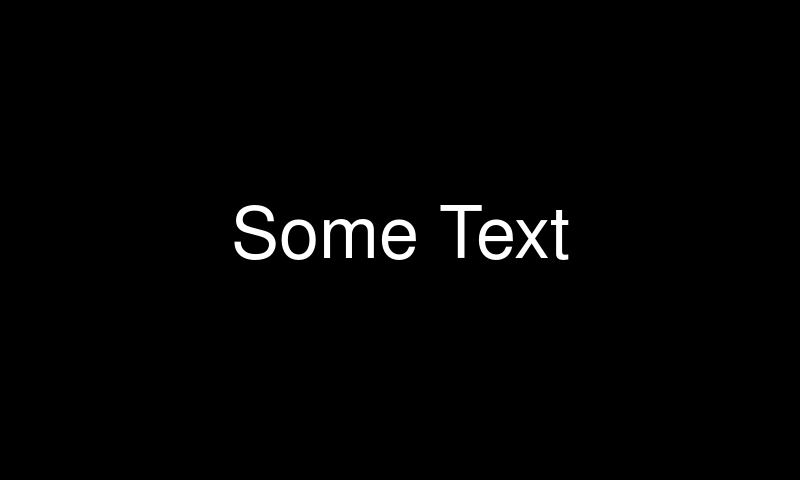
-background
– the background color for the image. This can be a hex value or a word such as pink.-size
– the dimensions for the image (widthxheight).-fill
– the color of the text.-pointsize
– the size of the text, play around with this value to get the desired result.-gravity
– where to place the text within the image.label:
– the text to write.
The final option is the output file. If you don't supply a path the image will be created in your current working directory.
Customising the Font
To use a custom font, first look up what fonts are available to use on your system using the following command:
convert -list font
To narrow the results down, pipe in grep
followed by an expression. Let's say we want to see what Helvetica fonts are available – we could use this command:
convert -list font | grep -i Helvetica
family: Helvetica
Font: Helvetica
family: Helvetica
Font: Helvetica-Bold
family: Helvetica
Font: Helvetica-BoldOblique
...
Now we can supply the name of the font to use with the -font
option.
convert -background "#000000" -size 800x480 -fill "#ffffff" -pointsize 72 -font "Helvetica-Bold" -gravity center label:'Some Text' output.png
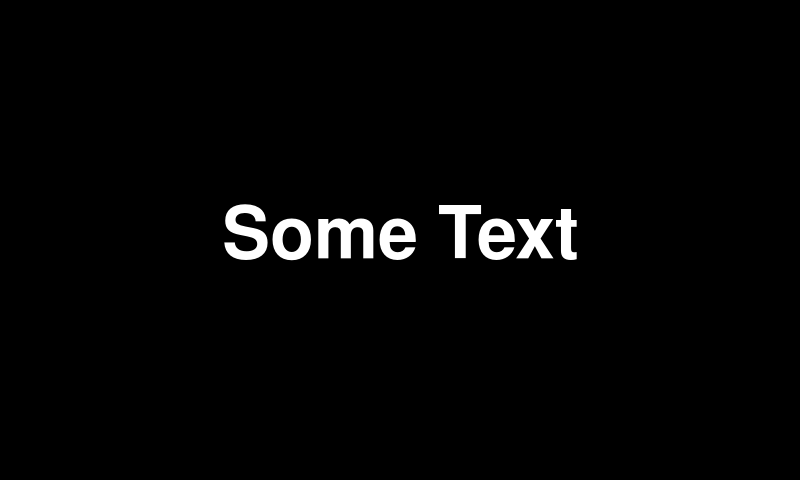
Generating Multiple Images in a PHP Program
Here is an example of generating images programatically in PHP with randomly selected background colors:
$images = ['a.png', 'b.png','c.png'];
foreach ($images as $key => $i) {
$output_path = public_path('images/thumbs/'. $i);
$colors = ['E4D870', '45AACB', 'F2BDCD', '473D89', '536877', 'DD143C', 'D38F61', '009E6C', '85618E', '788BA5', '00469A', '7E7275', '6A2A35', 'A7C3BC', '3D3986'];
$bg = '#'. $colors[rand(0, count($colors) -1)];
shell_exec('convert -background "'.$bg.'" -size 460x270 -fill "#ffffff" -font "Helvetica-Bold" -pointsize 25 -gravity center label:"'.$i.'" '. $output_path);
}